Using the AjaxControlToolKit AutoCompleteExtender
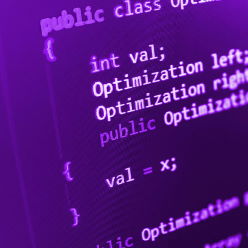
Option A: (the static page method option)
- Add a TextBox to your page
- Add the AutoCompleteExtender
- Set the ScriptManager property EnablePageMethods to true
- Add a static method to the page, one that gets a string and an integer as parameters and returns a string array
- Decorate it with [WebMethod(true/false)]. If you set the WebMethod parameter to true, it will have access to the Session
- Make sure to return the list of strings depending on the string parameter (which represents what was typed in the TextBox)
- Warning! If the method is faulty, you will get no error message, the autocomplete will simply not work.
- Warning! Having a different method signature will also cause this to not work.
- Set the properties for the AutoCompleteExtender: TargetControlID with the ID of the TextBox, MinimumPrefixLength with the count of typed characters from which to attempt autocomplete, ServiceMethod with the name of the static page method and CompletionInterval with the miliseconds before it attempts autocomplete.
Option B: (the web service option)
- Add a TextBox to your page
- Add the AutoCompleteExtender
- Add a webservice to your web site
- The webservice must have [ScriptService] decorating it's class in the codebehind
- Add a NOT static method to the webservice, one that gets a string and an integer as parameters and returns a string array
- Decorate it with [WebMethod(true/false)] and [ScriptMethod]. If you set the WebMethod parameter to true, it will have access to the Session
- Make sure to return the list of strings depending on the string parameter (which represents what was typed in the TextBox)
- Warning! If the method is faulty, you will get no error message, the autocomplete will simply not work.
- Warning! Having a different method signature will also cause this to not work.
- Set the properties for the AutoCompleteExtender: TargetControlID with the ID of the TextBox, MinimumPrefixLength with the count of typed characters from which to attempt autocomplete, ServiceMethod with the name of the WebMethod in the webservice, CompletionInterval with the miliseconds before it attempts autocomplete and ServicePath to the path of the asmx path.
Now it should work.
Code:
//=== AutoComplete.cs - the web service ===
[WebService(Namespace = "http://tempuri.org/")]
[WebServiceBinding(ConformsTo = WsiProfiles.BasicProfile1_1)]
[ScriptService]
public class AutoComplete : WebService
{
[WebMethod(true)]
[ScriptMethod]
public string[] GetList(string prefixText, int count)
{
string[] arr=new string[] {'list','of','words'};
return arr;
}
}
//=== Web page codebehind
[WebMethod(true)]
[ScriptMethod]
public static string[] GetList(string prefixText, int count)
{
string[] arr=new string[] {'list','of','words'};
return arr;
}
=== Web page ===
<asp:TextBox ID="textboxWithAutoComplete" runat="server">
<cc1:AutoCompleteExtender ID="autoCompleteExtender1" runat="server" TargetControlID="textboxWithAutoComplete"
MinimumPrefixLength="0"
ServiceMethod="GetList"
CompletionInterval="0"
ServicePath="AutoComplete.asmx"
>
</cc1:AutoCompleteExtender>
WARNING! The parameters of the web method must be named prefixText and count or the AutoCompleteExtender will NOT WORK!
A small paragraph that most people miss on the AjaxControlToolKit sample page says: Note that you can replace "GetCompletionList" with a name of your choice, but the return type and parameter name and type must exactly match, including case.