Styling Angular Material tooltips
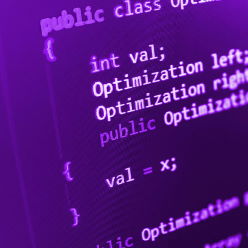
- Check whether myTooltipClass is defined in the component or the global CSS file. It should either be in the global CSS file (so it applies to everything) or your component should declare encapsulation: ViewEncapsulation.None
- Check the declaration of the class is specific enough. DO NOT use !important to fix this, although it would work. Try something like this: mat-tooltip-component .mat-tooltip.myTooltipClass {...}
To see if the class is applied, set the background-color property to red or something. If the class applies, you managed to define it correctly. If it applies partially, it's not specific enough. To change the width, use max-width. To make the tooltip wrap use white-space: wrap; and word-wrap: break-word;
As a reference, this is how the HTML looks for a rendered tooltip:
<div class="cdk-overlay-container">
<div class="cdk-overlay-connected-position-bounding-box" dir="ltr" style="top: 0px; left: 0px; height: 100%; width: 100%;">
<div id="cdk-overlay-1" class="cdk-overlay-pane mat-tooltip-panel" style="pointer-events: auto; top: 8px; left: 417.625px;">
<mat-tooltip-component aria-hidden="true" class="ng-tns-c34-15 ng-star-inserted" style="zoom: 1;">
<div class="mat-tooltip ng-trigger ng-trigger-state" style="transform-origin: left center; transform: scale(1);">Info about the action</div>
</mat-tooltip-component>
</div>
</div>
</div>