LInQer for Typescript is here!
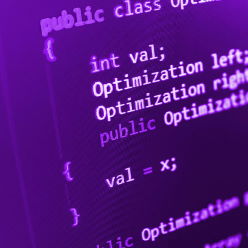
A year or so ago I wrote a Javascript library that I then ported (badly) to Typescript and which was adding the sweet versatility and performance of LINQ to the *script world. Now I've rewritten the entire thing into a Typescript library. I've abandoned the separation into three different Javascript files. It is just one having everything you need.
I haven't tested it in the wild, but you can get the new version here:
Github: https://github.com/Siderite/LInQer-ts
NPM: https://www.npmjs.com/package/@siderite/linqer-ts
Documentation online: https://siderite.github.io/LInQer-ts
Using the library in the browser directly: https://siderite.github.io/LInQer-ts/lib/LInQer.js and https://siderite.github.io/LInQer-ts/lib/LInQer.min.js
Please give me all the feedback you can! I would really love to hear from people who use this in:
- Typescript
- Node.js
- browser web sites
The main blog post URL for the project is still https://siderite.dev/blog/linq-in-javascript-linqer as the official URL for both libraries.
Have fun using it!