Creating a Web User Control in NET 2.0 that can be validated
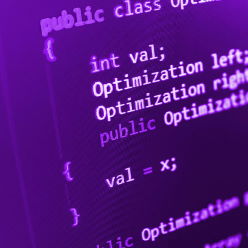
So, first step, you create your web user control by putting some controls in it. Then, you want to add a validator to the page to reference the newly created user control. And you get the error "Control '{0}' referenced by the ControlToValidate property of '{1}' cannot be validated.". Why? because every control to be validated needs to be decorated with the ValidationProperty attribute:
public partial class ucDate : System.Web.UI.UserControl
Adding the first line to the control tells the validation framework to use the Text property of the UserControl.
Next step, you run the page and you notice the javascript doesn't work. The client validation works on html controls, by looking (recursively) for a 'value' attribute. When one looks at the source code, though, there is no html control that has the id of the user control. It doesn't use a span or a div to encapsulate its controls. All the controls have the id to show they are children to the user control, but the actual user control does not appear in the html source. So what is there to do?
You put all the controls in the ascx file of the User Control into this div. There you go! The validation works!
There is one more quirk regarding web user controls that have more children that render an html object with a 'value' attribute. In that case, remember that the validation starts from the very top, in our case the div. One could build simple javascript functions on the onchange or onsubmit javascript events, for example, to add a value attribute to the div. Best way would be using the onsubmit event, but be careful that the validation sequence also runs on the onsubmit event.