Mobile operators transparently change the content you download from the Internet
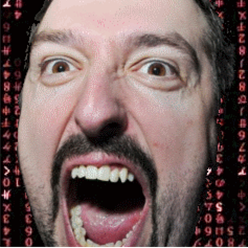
... and I don't mean something like injecting ads; I mean they modify the images you download and the pages that you read. They do that without telling you, under the umbrella of "improving your browsing experience". Let me give you some examples.
Today I copied two image files on a server: a JPEG and a PNG file. When downloaded via a normal network connection, I was getting the original file, about 50KB in size. When downloaded via 3G the image was different! In the jpeg case the file was smaller by a few hundred bytes and in the case of the PNG the file was actually bigger than the original. What was worse, the metadata information in those photos, like the software used to compress it, for example, was completely lost.
I couldn't believe my eyes. I strongly believe that what you ask for from the Internet you should get. This may not have been obvious for someone downloading the images in order to see them, but I was actually conducting a test that depended on the exact size of the file I was downloading. This practice seems to be widespread, but when Googling for it very few links pop up, showing that people mostly have no idea that it happens. In this operator's case, they seem to only change images, but people on the Internet tell stories of bundling CSS and Javascript files inside HTML files, or removing comments from either of them.
The morality of this is dubious at best, in my view it should be illegal, however things are worse than that: this behavior breaks functionality in existing sites. How can you possibly guarantee that your application works as expected when mobile operators (and I guess any ISP) can change your content arbitrarily and without the possibility to opt out? It's like that joke with the boyscout explaining at camp that little old ladies are hardier than one might think, as they squirm and shout and hit you when you try to cross them the street and the instructor soon finds out that it never occurred to the boy that he should first make sure they want to get to the other side.
Here are some links regarding this, just to make sure people can find them more easily:
Mobile operators altering (and breaking) web content
Should mobile operators be free to modify content they deliver?
Mobile Proxy Cache content modification by O2
O2 UK mobile users - your operator is breaking this site for you.
Prevent mobile website image compression over 3G
Get rid of image compression on O2′s network
ByteMobile Adaptive Traffic Management
From the last link you can see that they are caching and modifying even movies, through practices like giving you a lower rate movie or caching a version with a lower resolution. They do this in the name of delivering you video content compressed with a format and codec that your device can safely open.
A solution for this? Encryption. Using HTTPS prevents access to the content from a third party. HTTPS is becoming more and more used, as the hardware requirements for its implementation become less restrictive and with the many revelations about government scrutiny of Internet communications. However, you might be interested to know that mobile operators are feeling threatened by it. Articles from their point of view decry the "threat" of encryption and the solutions against it! The terrible impact of encryption is seen as an impediment in their rightful "content and delivery optimization techiques".
Things are getting even worse. Remember the concept of network neutrality? It is a very hot topic today and a very important political and economic fight is being fought right now to protect the transparency of the Internet. However, if you look further, you see that nobody considers the practice of "optimization" as something net neutrality should protect. In December 2010, the US Federal Communications Commission set three basic rules for net neutrality:
- Transparency. Fixed and mobile broadband providers must disclose the network management practices, performance characteristics, and terms and conditions of their broadband services;
- No blocking. Fixed broadband providers may not block lawful content, applications, services, or non-harmful devices; mobile broadband providers may not block lawful websites, or block applications that compete with their voice or video telephony services; and
- No unreasonable discrimination. Fixed broadband providers may not unreasonably discriminate in transmitting lawful network traffic.
So they are primarily focusing on blocking and throttling, but not on preserving the integrity of the transmitted data!