Flu: The Story Of the Great Influenza Pandemic of 1918 and the Search for the Virus That Caused It, by Gina Kolata
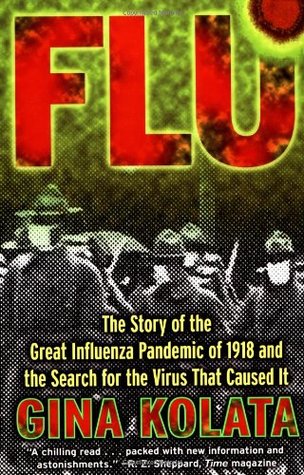
Gina Kolata's Flu: The Story of the Great Influenza Pandemic of 1918 starts with a question that, I believe, is more important than the cause of the epidemic or even its terrible effects: Why don't we know about what happened in 1918? Why isn't it taught at school, why isn't there more information about what happened, why aren't there more books, songs, reports, movies and memoirs about what can be safely called the biggest killer event in human history in terms of numbers? Not only regular people, but also medical professionals find themselves surprised to know so little about it. In fact, if we weren't living during pandemic times right now and the parallel hadn't been drawn, we would still be in a general state of ignorance about the very existence of the event.
And it's not only the military censorship during World War I, which surely had a lot to do with it, but a more insidious and yet generalized reason: people want to forget about it. The story is not one of heroism, it doesn't follow the hero's journey, it doesn't end with humanity defeating its foe, with suffering teaching an important lesson or with a patriarchal being or group coming to the rescue. Instead, it is one of utter defeat, of something unknown devastating tens of millions of lives, while they are incapable to do anything and no one comes to their aid or even acknowledges their suffering. Just senseless death, then it's over. Just like surviving a bear attack after the bear got bored of clawing and biting bits of your crying, self pissing helpless body.
Yet the rest of the book is about the unsung heroes of the story, scientists that attempt to learn from the experience and guard against it happening again. It follows people trying to find the infectious agent that caused the 1918 pandemic, identify it and pry its secrets. These are people that focus on a task and obsess about finding ways to complete it. They work tirelessly in labs, dig up century old frozen bodies to find what killed them, overcome obstacles (mostly put there by other people) and are the only ones who actually know anything about something and can guide action against future disease.
Gina Kolata's book is a great read in these times, because it not only shows people are actually doing something about epidemics, even if no one is excitedly talking about it in the media, but also shows how every pandemic follows a predictable path. It's not just our politicians that seem to be completely baffled on what to do. It's not just our scientists who get blocked in their work by malicious rumor and public opinion about things they understand nothing about. It's not just our industry that for financial reasons thwarts efforts to do something and not just us that think random chemicals can safeguard us from the new threat. Epidemics are the great stories that no one learns from and therefore we are all doomed to repeat living through them.
Flu is also a great companion to Barry's The Great Influenza book. While that one was more historical in nature and focused more on the path the virus took and how people reacted to it, Kolata is almost taking the reverse path, starting with current efforts to understand what happened in 1918 and thus slowly creating a picture of the events then, through the work of dedicated scientists and the biological information discovered about the virus.
Bottom line: this is a book well worth reading, particularly in these troubled times. Hell, it's not like you have a lot of other things to do anyway!