Editing HTML directly in your browser!
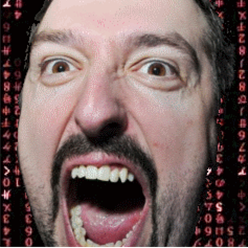
javascript:document.body.contentEditable='true'; document.designMode='on'; void(0);
Pasted into the address bar of browsers like IE6, IE7, FF2 (haven't tested on others, but I think it should work on most) it allows the editing of HTML directly on the page. You can expand and shrink HTML elements, you can edit text, etc.
While on Internet Explorer the changes are not saved if you save the page, on FireFox you get spell checking while editing (with red underlines for unrecognized words) and you can save the changes with save page.
It's really weird, check it out.
Bookmark AJAX pages.
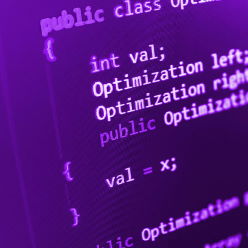
Javascript can modify and read this value with no refresh of the page. Also, if you have anchors in your page, like... <a name="hash" Javascript code like
window.location.hash='hash';will scroll the page there.
Javascript replace function
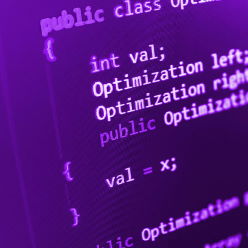
Simon Willison's Weblog contains a good article on this.
Basically, if you use str1.replace(str2,str3) it will return str1 with the first occurence of str2 replaced with str3. If you use str1.replace(regexp1,str3) and the regular expression has the g modifier it will return str1 with all matches of regexp1 replaced with str3.
A regular expression looks like : /searchpattern/modifiers.
You can create a regular expression from a string by using the
new RegExp(str2,modifiers)syntax.
The problem comes when you want to create a regular expression from a variable that may contain regular expression escape sequences. Here is Simon Willison's function that "escapes" the string in order to use the RegExp syntax safely, slightly modified to contain the '^' character:
RegExp.escape = function(text) {
if (!arguments.callee.sRE) {
var specials = [
'/', '.', '*', '+', '?', '<', '>',
'(', ')', '[', ']', '{', '}', '\\', '^'
];
arguments.callee.sRE = new RegExp(
'(\\' + specials.join('\\') + ')', 'g'
);
}
return text.replace(arguments.callee.sRE, '\\$1');
}
Example: str=str.replace(/\\/g,'\\\\') (replace slashes with double slashes)
Programatically trigger event in Javascript
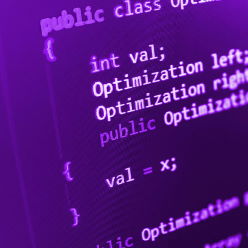
IE way) really simple, use element.fireEvent(eventName,[eventObject]), where element is a HTML element and eventName is something like "onclick";
DOM way) really complicated. A short example:
function simulateClick() {
var evt = document.createEvent("MouseEvents");
evt.initMouseEvent("click", true, true, window,
0, 0, 0, 0, 0, false, false, false, false, 0, null);
var cb = document.getElementById("checkbox");
var canceled = !cb.dispatchEvent(evt);
if(canceled) {
// A handler called preventDefault
alert("canceled");
} else {
// None of the handlers called preventDefault
alert("not canceled");
}
}
As you can see, you need to create the event, then initialize it, then dispatch it.
- Things you need to take care with:
- in the DOM model the event name is not prefixed with "on". In the IE way, the event is prefixed with "on". ex: DOM:click IE:onclick.
- in the DOM model the event triggering does everything the event would have done, like setting a value, in IE it does not. ex: triggering onclick in the above example would check or uncheck the box. In IE you need to change the value yourself.
This is code I did to programatically click a checkbox and also trigger the onclick event:
if (box.onclick)
{
if (box.dispatchEvent) {
var clickevent=document.createEvent("MouseEvents")
clickevent.initEvent("click", true, true)
box.dispatchEvent(clickevent)
} else
if (box.fireEvent) {
box.checked=!box.checked;
box.fireEvent('onclick');
} else
box.onclick();
} else box.checked=!box.checked;
Debugging Javascript with Visual Studio or other tools
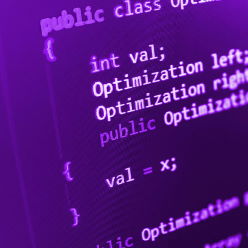
Basically, what needs to be done is first go to Internet Explorer Options and disable Disable Script Debugging (Internet Explorer) (No, I didn't mistype, there is a checkbox that needs disabling and it's called Disable...) in the Advanced section. Be careful not to disable the other script debugging box, only the Internet Explorer one. Then, when an error occurs, the system asks you what debugger to use. You can choose Visual Studio 2003 or 2005 from the list (provided you have them installed :) ), trace, put breakpoints, etc.
There is also the javascript debugger keyword, which fires a debugging window at the specific location, so it's like a breakpoint.
Googling the net I've found that there are both free and commercial Javascript debuggers. There is even a free one provided by Microsoft: Script Debugger for Windows. One, unfortunately shareware, product that I saw recommended on the forums is 1st JavaScript Editor.
The only disadvantage to this, that I've seen, is that any javascript error will result in opening the debuggers window, without the ability to check "ignore errors on this page" or anything like that. It could be annoying while browsing. Yet I am surprised that I've heard of this method of Javascript debugging only now.
Update:
If it seems the list of debuggers does not appear anymore (or never did), go to Visual Studio Tools -> Options -> Debugging -> Just-In-Time and check the "Script" checkbox.
If it seems Visual Studio doesn't correctly find the right Javascript line to debug when entering debug mode, start the web site without debugging and then choose Microsoft Script Debugger. It gets the current line right, but has a difficult to use interface (Hint: use the Command Window). Of course, you have to download and install it first :).
Add table header on each printed page
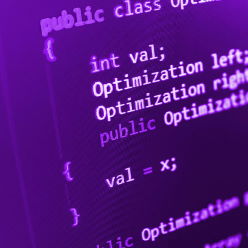
There is a simple solution for printing tables with repeating headers on each printed page. It involves CSS styling of the THEAD section of a table. Unfortunately, neither DataGrids nor GridViews render the THEAD tag. Somehow, Microsoft seems hellbent against it. So either create a control that renders THEAD, then add "display:table-header-group;" to the THEAD style, or use this Javascript function:
function AddTHEAD(tableName)
{
var table = document.getElementById(tableName);
if(table != null)
{
var head = document.createElement("THEAD");
head.style.display = "table-header-group";
head.appendChild(table.rows[0]);
table.insertBefore(head, table.childNodes[0]);
}
}
Update:Building a GridView, DataGrid or Table with THEAD, TBODY or TFOOT sections in NET 2.0
Cancel, kill, murder a javascript event
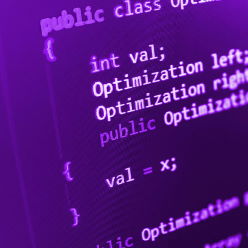
function murderEvent(evt) {
evt.cancel=true;
evt.returnValue=false;
evt.cancelBubble=true;
if (evt.stopPropagation) evt.stopPropagation();
if (evt.preventDefault) evt.preventDefault();
return false;
}
Internet Explorer and the hidden table cells.
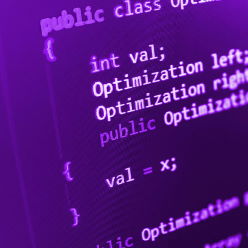
More detailed, style.display='none' makes the cellIndex of the next cells to decrement, while style.visibility='hidden' does not decrement the cellIndex, but keeps the formatting of the page, so you actually see a big empty space where the cell used to be.
I wouldn't have mind this if the Microsoft guys used another index to show the internal value, but they didn't! I have no idea what is the internal index of my cells anymore! Grrr!
Looping through Javascript object properties
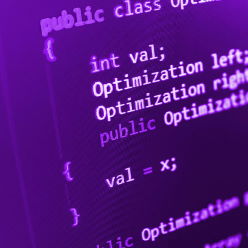
First of all, looping through the properties of a Javascript object:
// for ... in ...
for (var key in obj) {
console.log(key,obj[key]);
}
// using Object.keys (IE9+) and a normal for loop
var keys=Object.keys(obj);
for (var i=0; i<keys.length; i++) {
var key=keys[i];
console.log(key,obj[key]);
}
// using the Array.prototype.forEach function (IE9+)
Object.keys(obj).forEach(function(key) {
console.log(key,obj[key]);
});
// for ... of ... from the new ES6 specification
for (var key of Object.keys(obj)) {
console.log(key,obj[key]);
}
All of the methods above are similar, but not exactly the same. For example, the for...in loop goes over all the properties of an object, including those coming from its prototype. Object.keys (the other three methods, which all basically iterate over an array) only takes the set properties of the object itself. One can determine if an object has the property or it comes from the prototype using Object.prototype.hasOwnProperty and can determine if the object has a property of any kind using
var hasProperty = (typeof obj[key] != 'undefined')or the relatively more recent
var hasProperty = (obj[key] !== undefined)I say relatively more recent because in some browsers the value of undefined could be set. (Note the strict inequality sign, because undefined==null, but undefined!==null).
Now that we went through methods of iterating over the elements of arrays, it's trivial to enumerate the HTML attributes of an element, because they are stored in an attributes NamedNodeMap, which even if it looks like an array, with a length property and numeric indexes, it is not. forEach doesn't work, for example.
for (var i=0; i<obj.attributes.length; i++) {
var attr=obj.attributes[i];
console.log({
index:i,
name:attr.nodeName,
value:attr.nodeValue
});
}
Note that console.log(attr) will return a string like representation of the object because for some reason attributes have a valueOf overload that turns them into a string like key="value". You can also transform the NamedNodeMap into an array using Array.from (not in IE or Opera!) then use forEach, if that's your thing.
And below is the 2006 post... yuck!
custom controls. Imagine a tag like this:
<span id=test1 attribute1="value">
followed by a Javascript code like this:
test1.attribute2="anotherValue";
There are two solutions for reading the attributes of an object:
1. for-in loop:
for (var name in test1) alert(name+':'+test1[name]);
2. use the attributes property (the W3C DOM way)
for (var c=0; c<test1.attributes.length; c++) {
var attr=test1.attributes[c];
alert(attr.name+':'+attr.value);
}
Or, if you are fortunate enough to know the attribute name, you can use
obj.setAttribute(name,value);
value=obj.getAttribute(name);
How nice. Now the good part, these pieces of code work differently for IE
and non IE browsers. What a surprise.
IE:
- both loops show all the properties of an object, including methods,
attributes specified in JS code or in html tags. The functions specified in
a tag are returned as [object]s.
- also setAttribute and getAttribute work the same, returning object
properties or tag attributes
FireFox, Opera and Netscape:
- the for-in loop returns only the object properties and methods, NOT the
things defined in the HTML tag
- the attributes loop returns ONLY the tag attributes
- getAttribute will only return the tag attribute while setAttribute will
change only the attributes, not the object model
- in the case that a tag attribute is automatically transformed by the
browser in an object property (like an event onchange="test();")
obj.onchange will return a function object, while
obj.getAttribute('onchange') will return a string
Therefore the only way I found to loop through all the properties of both
html tag and DOM object is by using both loops. ex:
function PassAttr(elem1,elem2) {
for (var c=0; c<elem1.attributes.length; c++) {
var attr=elem1.attributes[c];
if (attr.name.substring(0,5)=='list_')
elem2.setAttribute(attr.name.substring(5),attr.value);
}
for (name in elem1)
if (name.substring(0,5)=='list_')
elem2.setAttribute(name.substring(5),elem1[name]);
}
The Microsoft Developer Toolbar
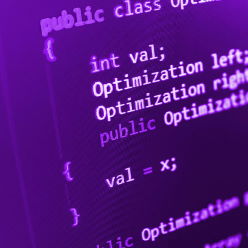
Internet Explorer Developer Toolbar
Then go in Internet Explorer and add the Developer toolbar. It is very nice
for web developers and javascript programmers
Also, check this out:
Code Spy
Weirdest javascript Date daylight savings bug
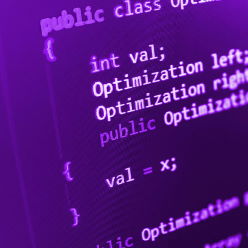
I found out that in Javascript, the Date object can give weird results.
Imagine that new Date(2005,2,27) [Javascript months are 0=11] returns 26 March 2005. If you look closely, you will see that it returns 26.03.2005 23:00:00.
Why? Because then our computers are configured to change the time with one hour. In a sense, this is not an error, as 27.03.2005 00:00:00 does not exist. You either have 1:00 of the 27th or 23:00 of the 26th. It is weird that Javascript both on IE and Netscape (and probably all other browsers) chooses the second option.
[Update thanks to a comment]I live in Romania. 27 of March 2005 is a daylight saving date (a bit non standard). Maybe the same bug can be reproduced for other countries on their respective daylight saving dates.[/Update]
This can yield weird results, as validators sometimes fail to recognize 27.03.2005 as a valid date. The solution for Javascript code: always use new Date(year, month, day, 12, 0, 0). The solution for validators... well, except calling Microsoft and bitching about it or changing the validator JS
file on ALL deployment servers, is either use a home made validator or replace the faulty validator js function in the pages that need date validation.
That means adding in the common site js this function:
function ValidatorConvert(op, dataType, val) {
function GetFullYear(year) {
return (year + parseInt(val.century)) - ((year < val.cutoffyear) ? 0 : 100);
}
var num, cleanInput, m, exp;
if (dataType == "Integer") {
exp = /^\s*[-\+]?\d+\s*$/;
if (op.match(exp) == null)
return null;
num = parseInt(op, 10);
return (isNaN(num) ? null : num);
}
else if(dataType == "Double") {
exp = new RegExp("^\\s*([-\\+])?(\\d+)?(\\" + val.decimalchar + "(\\d+))?\\s*$");
m = op.match(exp);
if (m == null)
return null;
cleanInput = m[1] + (m[2].length>0 ? m[2] : "0") + "." + m[4];
num = parseFloat(cleanInput);
return (isNaN(num) ? null : num);
}
else if (dataType == "Currency") {
exp = new RegExp("^\\s*([-\\+])?(((\\d+)\\" + val.groupchar + ")*)(\\d+)"
+ ((val.digits > 0) ? "(\\" + val.decimalchar + "(\\d{1," + val.digits + "}))?" : "")
+ "\\s*$");
m = op.match(exp);
if (m == null)
return null;
var intermed = m[2] + m[5] ;
cleanInput = m[1] + intermed.replace(new RegExp("(\\" + val.groupchar + ")", "g"), "") + ((val.digits > 0) ? "." + m[7] : 0);
num = parseFloat(cleanInput);
return (isNaN(num) ? null : num);
}
else if (dataType == "Date") {
var yearFirstExp = new RegExp("^\\s*((\\d{4})|(\\d{2}))([-/]|\\. ?)(\\d{1,2})\\4(\\d{1,2})\\s*$");
m = op.match(yearFirstExp);
var day, month, year;
if (m != null && (m[2].length == 4 || val.dateorder == "ymd")) {
day = m[6];
month = m[5];
year = (m[2].length == 4) ? m[2] : GetFullYear(parseInt(m[3],10))
}
else {
if (val.dateorder == "ymd"){
return null;
}
var yearLastExp = new RegExp("^\\s*(\\d{1,2})([-/]|\\. ?)(\\d{1,2})\\2((\\d{4})|(\\d{2}))\\s*$");
m = op.match(yearLastExp);
if (m == null) {
return null;
}
if (val.dateorder == "mdy") {
day = m[3];
month = m[1];
}
else {
day = m[1];
month = m[3];
}
year = (m[5].length == 4) ? m[5] : GetFullYear(parseInt(m[6], 10))
}
month -= 1;
var date = new Date(year, month, day,12,0,0); //BUG FIX
return (typeof(date) == "object" && year == date.getFullYear() && month == date.getMonth() && day == date.getDate()) ? date.valueOf() : null;
}
else {
return op.toString();
}
}