Learning ASP.Net MVC - Intermezzo - Upgrading to .Net Core 1.1
It is about time to revisit my series on ASP.Net MVC Core. From the time of my last blog post the .Net Core version has changed to 1.1, so just installing the SDK and running the project was not going to work. This post explains how to upgrade a .Net project to the latest version.
Learning ASP.Net MVC series:
Pressing the batch Update button for NuGet packages corrupted project.json. Here are the steps to successfully migrate a .Net Core project to a higher version.
Code after changes can be found on GitHub
That should do it. For detailed steps of what I actually did to get to this concise list, read on.
Downloaded the source code from GitHub, loaded the .sln with Visual Studio 2015. Got a nice blocking alert, because this was a .NET Core virgin computer:
Of course, I could have tried to install that version, but I wanted to upgrade to the latest Core.
And here I went to Announcing the Fastest ASP.NET Yet, ASP.NET Core 1.1 RTM. I followed the instructions there, made Visual Studio 2015 load my project and automatically restore packages:
I got to the second step, but still got the alert...
... so I commented out the sdk property in global.json. I got another alert:

This answer recommended uninstalling old versions of SDKs, in my case "Microsoft .NET Core 1.0.1 - SDK 1.0.0 Preview 2-003131 (x64)". Don't worry, it didn't work. More below:
TL;DR; version: do not uninstall the Visual Studio .NET Core Tooling
And then... got the same
I created a new .NET core project, just to see the version of SDK it uses: 1.0.0-preview2-003131 and I added it to global.json and reopened the project. It restored packages and didn't throw any errors! Dude, it even compiled and ran! But now I got a System.ArgumentException:
The third step in the Microsoft instructions was removed by me because it caused some problems to me. So don't do it, yet. It was
Since I had not upgraded the packages, as in the Microsoft third step, I decided to do it. 26 updates waited for me, so I optimistically selected them all and clicked Update. Of course, errors! One popped up as more interesting:
Suspecting it was a problem with the NuGet package manager, I went to the link in the first error. But in Visual Studio 2015 NuGet is included and it was clearly the latest version. So the only solution was to go through each package and see which causes the problem. And I went to 26 packages and pressed Install on each and it worked. Apparently, the batch Update button is causing the issue. Weirdly enough there are two packages that were installed, but remained in the Update tab and also appeared in the Consolidate tab: BundleMinifier.Core and Microsoft.EntityFrameworkCore.Tools, although I can't to anything with them there.
Another package (Microsoft.VisualStudio.Web.CodeGeneration.Tools 1.0.0) caused another confusing error:
So I tried to build the project only to be met with yet another project.json corruption error:
It compiled. It worked.
Learning ASP.Net MVC series:
- Setup
- MVC Concepts
- Authentication
- Entity Framework Fundamentals
- Upgrading project to .NET Core 1.1
- Dependency Injection and Services
Short version
Pressing the batch Update button for NuGet packages corrupted project.json. Here are the steps to successfully migrate a .Net Core project to a higher version.
- Download and install the .NET Core 1.1 SDK
- Change the version of the SDK in global.json - you can find out the SDK version by creating a new .Net Core project and checking what it uses
- Change "netcoreapp1.0" to "netcoreapp1.1" in project.json
- Change Microsoft.NETCore.App version from "1.0.0" to "1.1.0" in project.json
- Add
"runtimes": {
to project.json
"win10-x64": { }
}, - Go to "Manage NuGet packages for the solution", to the Update tab, and update projects one by one. Do not press the batch Update button for selected packages
- Some packages will restore, but remain in the list. Skip them for now
- Whenever you see a "downgrade" warning when restoring, go to those packages and restore them next
- For packages that tell you to upgrade NuGet, ignore them, it's an error that probably happens because you restore a package while the previous package restoring was not completed
- For the remaining packages that just won't update, write down their names, uninstall them and reinstall them
Code after changes can be found on GitHub
That should do it. For detailed steps of what I actually did to get to this concise list, read on.
Long version
Step 0 - I don't care, just load the damn project!
Downloaded the source code from GitHub, loaded the .sln with Visual Studio 2015. Got a nice blocking alert, because this was a .NET Core virgin computer:
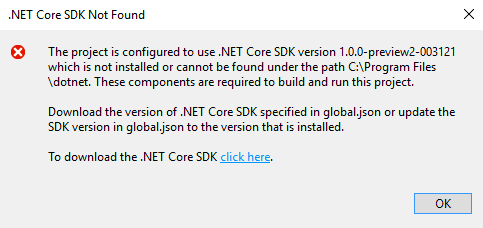
Step 1 - read the Microsoft documentation
And here I went to Announcing the Fastest ASP.NET Yet, ASP.NET Core 1.1 RTM. I followed the instructions there, made Visual Studio 2015 load my project and automatically restore packages:
- Download and install the .NET Core 1.1 SDK
- If your application is referencing the .NET Core framework, your should update the references in your project.json file for netcoreapp1.0 or Microsoft.NetCore.App version 1.0 to version 1.1. In the default project.json file for an ASP.NET Core project running on the .NET Core framework, these two updates are located as follows:
- to be continued...
I got to the second step, but still got the alert...
Step 2 - fumble around
... so I commented out the sdk property in global.json. I got another alert:
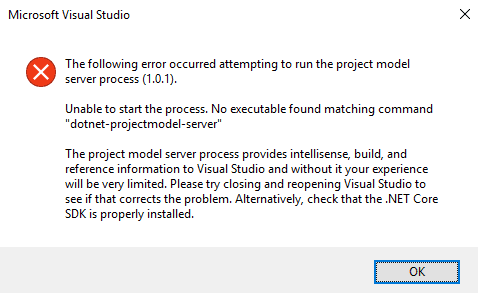
This answer recommended uninstalling old versions of SDKs, in my case "Microsoft .NET Core 1.0.1 - SDK 1.0.0 Preview 2-003131 (x64)". Don't worry, it didn't work. More below:
TL;DR; version: do not uninstall the Visual Studio .NET Core Tooling
And then... got the same
No executable found matching command "dotnet=projectmodel-server"error again.
I created a new .NET core project, just to see the version of SDK it uses: 1.0.0-preview2-003131 and I added it to global.json and reopened the project. It restored packages and didn't throw any errors! Dude, it even compiled and ran! But now I got a System.ArgumentException:
The 'ClientId' option must be provided.Probably it had something to do with the Secret Manager. Follow the steps in the link to store your secrets in the app. It then worked.
Step 1.1 (see what I did there?) - continue to read the Microsoft documentation
The third step in the Microsoft instructions was removed by me because it caused some problems to me. So don't do it, yet. It was
- Update your ASP.NET Core packages dependencies to use the new 1.1.0 versions. You can do this by navigating to the NuGet package manager window and inspecting the “Updates” tab for the list of packages that you can update.
Since I had not upgraded the packages, as in the Microsoft third step, I decided to do it. 26 updates waited for me, so I optimistically selected them all and clicked Update. Of course, errors! One popped up as more interesting:
Package 'Microsoft.Extensions.SecretManager.Tools 1.0.0' uses features that are not supported by the current version of NuGet. To upgrade NuGet, see http://docs.nuget.org/consume/installing-nuget.Another was even more worrisome:
Unexpected end of content while loading JObject. Path 'dependencies', line 68, position 0in project.json. Somehow the updating operation for the packages corrupted project.json! From a 3050 byte file, it now was 1617.
Step 3 - repair what the Microsoft instructions broke
Suspecting it was a problem with the NuGet package manager, I went to the link in the first error. But in Visual Studio 2015 NuGet is included and it was clearly the latest version. So the only solution was to go through each package and see which causes the problem. And I went to 26 packages and pressed Install on each and it worked. Apparently, the batch Update button is causing the issue. Weirdly enough there are two packages that were installed, but remained in the Update tab and also appeared in the Consolidate tab: BundleMinifier.Core and Microsoft.EntityFrameworkCore.Tools, although I can't to anything with them there.
Another package (Microsoft.VisualStudio.Web.CodeGeneration.Tools 1.0.0) caused another confusing error:
Package 'Microsoft.VisualStudio.Web.CodeGeneration.Tools 1.0.0' uses features that are not supported by the current version of NuGet. To upgrade NuGet, see http://docs.nuget.org/consume/installing-nuget.Yet restarting Visual Studio led to the disappearance of the CodeGeneration.Tools error.
So I tried to build the project only to be met with yet another project.json corruption error:
Can not find runtime target for framework '.NETCoreAPP, Version=v1.0' compatible with one of the target runtimes: 'win10-x64, win81-x64, win8-x64, win7-x64'. Possible causes: [blah blah] The project does not list one of 'win10-x64, win81-x64, win7-x64' in the 'runtimes' [blah blah]. I found the fix here, which was to add
"runtimes": {to project.json.
"win10-x64": { }
},
It compiled. It worked.
Comments
Be the first to post a comment