A really good programmer interview joke
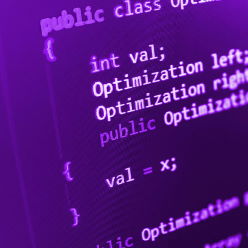
Here it is: What would Feynman do?
/// <summary>
/// Helper for Image objects
/// </summary>
public static class ImageHelper
{
/// <summary>
/// Get an image from a byte array
/// </summary>
/// <param name="iconBytes"></param>
/// <returns></returns>
public static Image GetImageFromBytes(byte[] iconBytes)
{
if (iconBytes == null || iconBytes.Length == 0)
return null;
using (MemoryStream ms = new MemoryStream(iconBytes))
{
try
{
return GetImageFromStream(ms);
}
catch
{
return null;
}
}
}
/// <summary>
/// Get an image from a file without locking the file
/// </summary>
/// <param name="fileName"></param>
/// <returns></returns>
public static Image GetImageFromFile(string fileName)
{
try
{
using (var stream = File.Open(fileName, FileMode.Open, FileAccess.Read, FileShare.ReadWrite))
{
return GetImageFromStream(stream);
}
}
catch
{
return null;
}
}
/// <summary>
/// Determines if an image is indexed (with a color palette)
/// </summary>
/// <param name="image"></param>
/// <returns></returns>
public static bool IsImageIndexed(Image image)
{
switch (image.PixelFormat)
{
case PixelFormat.Format1bppIndexed:
case PixelFormat.Format4bppIndexed:
case PixelFormat.Format8bppIndexed:
case PixelFormat.Indexed:
return true;
}
return false;
}
/// <summary>
/// Get an image from stream without locking the stream
/// </summary>
/// <param name="stream"></param>
/// <returns></returns>
public static Image GetImageFromStream(Stream stream)
{
try
{
using (var image = Image.FromStream(stream))
{
var srcBitmap = image as Bitmap;
var destBitmap = new Bitmap(image.Width, image.Height,image.PixelFormat);
if (IsImageIndexed(image)&&srcBitmap!=null)
{
Rectangle srcArea = new Rectangle(0, 0, image.Width, image.Height);
BitmapData srcData = srcBitmap.LockBits(srcArea, ImageLockMode.ReadOnly, image.PixelFormat);
Rectangle destArea = new Rectangle(0, 0, image.Width, image.Height);
BitmapData destData = destBitmap.LockBits(destArea, ImageLockMode.WriteOnly, image.PixelFormat);
IntPtr srcPtr = srcData.Scan0;
IntPtr destPtr = destData.Scan0;
byte[] buffer = new byte[srcData.Stride*image.Height];
Marshal.Copy(srcPtr, buffer, 0, buffer.Length);
Marshal.Copy(buffer, 0, destPtr, buffer.Length);
srcBitmap.UnlockBits(srcData);
destBitmap.UnlockBits(destData);
destBitmap.Palette = srcBitmap.Palette;
} else
{
using (var graphics = Graphics.FromImage(destBitmap))
{
graphics.DrawImage(image, 0, 0);
}
}
return destBitmap;
}
}
catch
{
return null;
}
}
}
You probably wonder why I haven't used jQuery. It is because I want it to work in a javascript: url so I can put it in a bookmark! Just as with the Firebug bookmark you need to manually create a bookmark in the Favorites folder (or to add any page as a favorite, then edit its URL) in Internet Explorer or to simply add a bookmark in the browser in Chrome and Firefox and paste the one line script. To make it easier, I will write them both here. The content of the .url favorite file:
var trs=document.getElementsByTagName('tr');
var l=trs.length;
for (var i=0; i<l; i++) {
var tr=trs[i];
var data=tr.getAttribute('data');
if (!data||data.indexOf('inherited')==-1) continue;
tr.style.display=tr.style.display=='none'?'':'none';
}
[DEFAULT]and the javascript line to use in other browsers:
BASEURL=http://msdn.microsoft.com/en-us/library/
[InternetShortcut]
URL=javascript:var trs=document.getElementsByTagName('tr');var l=trs.length;for (var i=0; i<l; i++) { var tr=trs[i]; var data=tr.getAttribute('data'); if (data&&data.indexOf('inherited')>-1) tr.style.display=tr.style.display=='none'?'':'none'; }; void(0);
IDList=
IconFile=http://msdn.microsoft.com/favicon.ico
IconIndex=1
[{000214A0-0000-0000-C000-000000000046}]
Prop3=19,2
javascript:var trs=document.getElementsByTagName('tr');var l=trs.length;for (var i=0; i<l; i++) { var tr=trs[i]; var data=tr.getAttribute('data'); if (data&&data.indexOf('inherited')>-1) tr.style.display=tr.style.display=='none'?'':'none'; }; void(0);.
The latest sources are now on Github: C# 4.0 library to generate INotifyPropertyChanged proxy from POCO type. The source from the post, designed as a proof of concept, is not the same as the one from Github.
It all started from a Sacha Barber post on CodeProject, enumerating ways in which one can use Aspect Oriented Programming to mark simple automatic properties so that they get compiled into fully fledged INotifyPropertyChanged properties, thus saving us the grief of repeating the same code over and over again in each property setter. The implementations there were good, but too complex, relying on third party libraries, some of them not even free.
He completely ignored template generators like T4, but then again, that particular approach has a lot of issues associated with it, like having to either parse source files or somehow tap into the compiled assembly... before you compile it.
However, this brought forth the idea that I could do this, maybe in some other way.
Enter Felice Pollano, with his article on CodeProject detailing a method of using CodeDom generation to create at runtime the INotifyPropertyChanged object from an already existing type. This is pretty slow, but only when first creating the type, so with a cache system it would be totally usable. I liked this approach better, but I noticed there were some errors in the generated code and when I tried changing the generating code I had to look at it for half an hour just to understand where to change it. Wouldn't it be better to use some sort of template that would be easy to edit and use it to generate the proxy type?
So this is my take on generating INotifyPropertyChanged classes dynamically, avoiding the repetitive plumbing in property setters. The library contains a template for the class and a separate template for the properties. The proxy type is being generated in memory from a string that is generated from the source type and the two templates. All in all, one class, two templates, three public methods and four static methods. As easy as 1,2,3,4 :) Here is the code:
As you can see, the code needs only a type that has public virtual properties in it and it will create a proxy that will inherit that class, implement INotifyPropertyChange and override each virtual property with a notifying one. The templates are so basic that I feel slightly embarrassed; I keep thinking if I should have created template entities that would stay in the same file. :) Here are the templates:
{usings}
namespace __generatedINotifyPropertyChanged
{
public class {className} : {baseClassName},INotifyPropertyChanged
{
public event PropertyChangedEventHandler PropertyChanged;
{properties}
private void OnPropertyChanged(string propertyName)
{
var handler=PropertyChanged;
if (handler != null)
{
handler(this, new PropertyChangedEventArgs(propertyName));
}
}
}
}
public override {propertyType} {propertyName}
{
get { return base.{propertyName}; }
set {
if (!Equals(value,base.{propertyName})) {
base.{propertyName}=value;
OnPropertyChanged("{propertyName}");
}
}
}
Don't forget to save the templates as Embedded Resource in the assembly.
Update: At Sacha Barber's advice I took a look at the DynamicObject solution for the INotifyPropertyChanged code smell. The link he provided is OlliFromTor's CodeProject article Using DynamicObject to Implement General Proxy Classes. While this works a lot easier than with compiling generated code, it also has a major drawback: the proxy class does not inherit from the original object and so it can only be used as such, but only in dynamic scenarios. Otherwise, it seems to be a perfect solution for proxy scenarios, if you are willing to discard the type safety.
Event Type: ErrorI did the classic
Event Source: ASP.NET 4.0.30319.0
Description:
aspnet_wp.exe could not be started. The error code for the failure is C0000142. This error can be caused when the worker process account has insufficient rights to read the .NET Framework files. Please ensure that the .NET Framework is correctly installed and that the ACLs on the installation directory allow access to the configured account.
iisreset /stopto no avail. I was about to curse and restart the computer again when I found this pretty little link: IIS doesn't start. Error code: C0000142. A solution is at the bottom, as the least voted answer: Go to Task Manager, kill explorer.exe, Run another explorer.exe. This starts IIS (aspnet_wp.exe under inetinfo.exe) correctly.
%windir%\Microsoft.NET\Framework\v4.0.30319\aspnet_regiis.exe -i
iisreset /start
[DOC_FirebugUI]then save it in the user's c:\Documents and Settings\{UserName}\Favorites folder.
ORIGURL=about:blank
[{000214A0-0000-0000-C000-000000000046}]
Prop3=19,2
[InternetShortcut]
URL=javascript:var script=document.createElement('script'); script.type='text/javascript'; script.src='https://getfirebug.com/firebug-lite.js';document.getElementsByTagName('body')[0].appendChild(script); void(0);
IDList=
IconFile=http://getfirebug.com/img/favicon.ico
IconIndex=1
javascript:var script=document.createElement('script'); script.type='text/javascript'; script.src='https://getfirebug.com/firebug-lite.js';document.getElementsByTagName('body')[0].appendChild(script); void(0);
When I first tried to do drag and drop in WPF I thought it would be something easy like DragAndDrop.IsDraggable="True". Boy,was I wrong! The mechanism for this has remained almost unchanged from the Windows Forms version. It is completely event driven and has nothing in the way of actual graphical feedback of what is going on except making the mouse cursor look different. If you want to do it using MVVM, you have another thing coming.
Disclaimer:Now, I have found a good solution for all the problems above, but the drag and drop behaviour is part of a larger framework that I have been working on and creating a separate project just for it might prove difficult. I mean, you want to show MVVM drag and drop, you should also have Views and ViewModels and base classes and helpers and everything. The solution, I guess, is to make separate articles for the main features in the framework, then present the project as a whole in the end. The framework itself is work in progress and untested in a real life project, so this might have to wait, as well. I will make sure, though, to put much code directly in this post.
First a bit of thanks to the people that inspired me to work on that. I have Sascha Barber to thank for his comprehensive articles on WPF, especially the ones about his Cinch framework. Then Lee Roth and Bea Stollnitz/Costa for the ideas about using adorners to show the drag and drop items. Finally Jason Young, for writing about MVVM Drag and Drop, but without the graphic part.
Ok then, let's define the requirements of this system. We need:
From these requirements we can form a basic idea of the way we would like this to work. First of all, we need the ability to mark any element as a drag item. Also, we need a container that can be marked as a drop target. We can do this using boolean IsDragSource and IsDropTarget Attached Properties; once set they will force a bind of the drag and drop events to some special handlers that would then direct decisions to ICommands.
As we are doing it in MVVM, we don't use the elements directly, but the data they represent, so we work with dragging and dropping commands using data objects. The classes responsible with the decisions for the drop permissions and actions should be in the ViewModel. We could, of course, link all events to commands, but that would be very cumbersome to use. Besides, we want it simple, we don't really want the user of the system to care about the drag and drop inner workings. Therefore, the solution is to change the IsDragSource and IsDropTarget to DragSource and DropTarget properties that accept objects of type IDragSource and IDropTarget containing all the methods needed for the events in question:
/// <summary>
/// Holds the data of a dragged object in a drag-and-drop operation.
/// </summary>
public interface IDraggedData
{
/// <summary>
/// A dictionary with the format as the key and the data in that format in the value
/// </summary>
IDictionary<string, object> Values
{
get;
}
/// <summary>
/// Optional object for additional information
/// </summary>
object Tag
{
get;
}
}
/// <summary>
/// Business end of the drag source
/// </summary>
public interface IDragSource
{
/// <summary>
/// Gets the supported drop effects.
/// </summary>
/// <param name="dataContext">The data context.</param>
/// <returns></returns>
DragEffects GetDragEffects(object dataContext);
/// <summary>
/// Gets the data.
/// </summary>
/// <param name="dataContext">The data context.</param>
/// <returns></returns>
object GetData(object dataContext);
}
/// <summary>
/// Defines the handler object of a drop operation
/// </summary>
public interface IDropTarget
{
/// <summary>
/// Gets the effects.
/// </summary>
/// <param name="dataObject">The data object.</param>
/// <returns></returns>
DragEffects GetDropEffects(IDraggedData dataObject);
/// <summary>
/// Drops the specified data object
/// </summary>
/// <param name="dataObject">The data object.</param>
void Drop(IDraggedData dataObject);
}
We need the methods for the effects to instruct the system about the types of operations that are allowed during drag and drop: None, Copy, Move, Scroll, All.
If you are going for the purist approach, you should use your own DragEffects enumeration, as above, since the DragDropEffects enumeration is in the System.Windows assembly, which in theory should have nothing to do with the ViewModel part of the application (one could want to use the ViewModel in a web environment, for example, or in a console application).
You will notice that the GetDropEffects method receives an IDraggedData object. This is also because the IDataObject interface and the DataObject class used in Windows drag and drop operations are also in the System.Windows assembly.
The IDraggedData interface is basically a dictionary that uses the data format as the key and the dragged data object stored in that format as the value. An important fact is that, when trying to drag and drop between applications, you need that the dragged data object be binary serializable. If not, you will only get the expected result when dragging to the same application. Here is an implementation of the interface, complete with a totally lazy way of getting the data based on which type is more "important":
/// <summary>
/// Holds data for a drag-and-drop operation
/// </summary>
public class DraggedData : IDraggedData
{
#region Instance fields
private Dictionary<string, Exception> mExceptions;
private Dictionary<string, object> mValues;
#endregion
#region Properties
/// <summary>
/// A dictionary with the format as the key and the data in that format in the value
/// </summary>
/// <value></value>
public Dictionary<string, object> Values
{
get
{
if (mValues == null)
{
mValues = new Dictionary<string, object>();
}
return mValues;
}
}
/// <summary>
/// A dictionary with the format as the key and the data in that format in the value
/// </summary>
/// <value></value>
IDictionary<string, object> IDraggedData.Values
{
get
{
return Values;
}
}
/// <summary>
/// Optional object for additional information
/// </summary>
/// <value></value>
public object Tag
{
get;
set;
}
/// <summary>
/// A dictionary for exceptions when retrieving the data in a specified format
/// </summary>
/// <value>The exceptions.</value>
public Dictionary<string, Exception> Exceptions
{
get
{
if (mExceptions == null)
{
mExceptions = new Dictionary<string, Exception>();
}
return mExceptions;
}
}
#endregion
}
In the IDragSource interface we need the GetData method to extract the data object associated with a dragged object, since the Windows drag and drop mechanism encapsulates the objects in an application agnostic way, so one can perform drag and drop between applications or to/from the operating system. Finally, we need the Drop method in the IDropTarget interface to handle in the ViewModel what happends when an item is dropped.
Let's get to the juicy part: a DragService static class that will register the attached properties that we need. Besides the DragSource and DropTarget properties we need status properties like DragOverStatus (for the target), DraggedStatus and IsDragged (for the original dragged item) as well as two properties called BringIntoViewOnDrag and ActivateOnDrag which would bring an item completely into view or activate it (if a Window) when a valid drop target. This one is long. It also contains some extension methods that would be explained later.
Most of the code here is self explanatory: you have attached property that bind to the element drag and drop events and handle them either through direct code or by delegating to the values set. There are some extension methods like ExecuteWhenLoaded and ExecuteWhenUnloaded which execute an action when the element has finished loading or when it is unloading. An important aspect here is that a window closing does not trigger the Unloaded event for its child elements, so you need to bind to the Dispatcher.StartedShutdown event as well:
/// <summary>
/// Execute an action when an element is unloaded
/// </summary>
/// <param name="element"></param>
/// <param name="action"></param>
public static void ExecuteWhenUnloaded(this UIElement element, Action action)
{
if (element == null)
{
throw new ArgumentNullException("element",
Resources.
UIExtensions_ExecuteWhenUnloaded_Element_cannot_be_null);
}
RoutedEventHandler elementOnUnloaded = null;
EventHandler dispatcherOnShutdownStarted = null;
// ReSharper disable AccessToModifiedClosure
elementOnUnloaded = (sender, args) =>
{
performExecution(element,
dispatcherOnShutdownStarted,
elementOnUnloaded,
action);
};
dispatcherOnShutdownStarted = (sender, args) =>
{
performExecution(element,
dispatcherOnShutdownStarted,
elementOnUnloaded, action);
};
// ReSharper restore AccessToModifiedClosure
FrameworkElement frameworkElement = element as FrameworkElement;
if (frameworkElement != null)
{
frameworkElement.Unloaded += elementOnUnloaded;
}
element.Dispatcher.ShutdownStarted += dispatcherOnShutdownStarted;
}
That is pretty much it for the drag and drop itself. You can implement IDropTarget on the ViewModel directly, but IDragSource needs to be binary serializable if you intend to drag and drop across application domains, so you usually implement it into a separate class that is a property of the dragged item view model or DataContext.
Because the DragService sets the status properties for each element, you can manipulate the appearance and behaviour of both drag source and drop target based on them. However, at this point the mouse will be the only indication that you are dragging something. You might want to actually drag something, especially since in a move operation the original element would be hidden during the drag. The problem here is that the drag source element cannot control the display of the dragged item in other applications, nor should it in its application, since it is not its responsibility. The solution: decorate an element over which you would drag something (like the root element of the entire window) with something that knows how to display dragged items:
This code uses an adorner to display the dragged item over it. Nothing fancy, since the actual template for the dragged data is defined in the decorator as the content. This is not the place to discuss the adorner, though, so here is just the code:
You would only need to decorate a view and then define the AdornerContent property for the decorator and, optionally, the grab point offset for the dragged element.
All that is left here is to show some usage examples. Let's assume we need a View over which we can drag and drop items:
<UserControl x:Class="BestPractices.Views.SecondaryView"
[...]
UIUtils:DragService.DropTarget="{Binding .}"
UIUtils:DragService.BringIntoViewOnDrag="True"
UIUtils:DragService.ActivateOnDrag="True"
UIUtils:DragService.DragOverStatus="{Binding DragOverStatus,Mode=OneWayToSource}">
Here you have a user control view which has the drop target set to its own view model and it is set to update the DragOverStatus property of the ViewModel when its attached DragOverStatus property is changed. The status properties are inheritable, so all the children of the view have them set. It is easy to define a Button style that has its text bolded when a copy operation is allowed for a drop item:
<Style TargetType="{x:Type Button}" BasedOn="{StaticResource {x:Type Button}}" > <Style.Triggers>
<DataTrigger Binding="{Binding DragOverStatus}" Value="{x:Static Utils:DragEffects.Copy}">
<Setter Property="FontWeight" Value="Bold"/>
</DataTrigger>
</Style.Triggers
></Style>
Its content is more interesting:
<UIUtils:DragAndDropAdornerDecorator Offset="40,40">
<UIUtils:DragAndDropAdornerDecorator.AdornerContent>
<Controls:ContentItem
DataContext="{Binding Path=(UIUtils:DragAndDropAdornerDecorator.DraggedData),
RelativeSource={RelativeSource Self}}"
Opacity="0.7"/>
</UIUtils:DragAndDropAdornerDecorator.AdornerContent>
<DockPanel Background="{Binding Background,
RelativeSource={RelativeSource AncestorType={x:Type UserControl}}}">
<Button Command="{m:CommandBinding AddItemCommand}"
DockPanel.Dock="Top"
>Add item</Button>
<Button Command="{m:CommandBinding RemoveItemCommand}"
DockPanel.Dock="Top"
>Remove item</Button>
<WrapPanel x:Name="ContainerPanel" >
</WrapPanel>
</DockPanel>
</UIUtils:DragAndDropAdornerDecorator>
The container for the items is a simple WrapPanel and it is placed in a dock panel together with add and remove item buttons. This dock panel is decorated as a drag visual container, and the content that is dragged is set to a custom control called ContentItem, with a drag point set to 40,40. The DataContext property of the item is set to the DraggedData property so that it expresses the actual dragged object.
Now we have set up a container to be a drop target for items. It displays the items as they are dragged over it. All we have left is to set up the items, the ContentItem control, to be a DragSource:
<Style TargetType="{x:Type Controls:ContentItem}">
<Setter Property="UIUtils:DragService.DragSource" Value="{Binding DragSource}"/>
<Setter Property="UIUtils:DragService.IsDragged" Value="{Binding IsDragged,Mode=OneWayToSource}"/>
<Setter Property="UIUtils:DragService.DragStatus" Value="{Binding DragStatus,Mode=OneWayToSource}"/>
<Setter Property="Background" Value="LightBlue"/>
<Setter Property="Template">
<Setter.Value>
<ControlTemplate TargetType="{x:Type Controls:ContentItem}">
<Border BorderThickness="1" BorderBrush="Blue" CornerRadius="2" Background="{TemplateBinding Background}" Width="75" Height="60">
<TextBlock Text="{Binding Id}" HorizontalAlignment="Center" VerticalAlignment="Center" FontWeight="Bold" FontSize="22"/>
</Border>
</ControlTemplate>
</Setter.Value>
</Setter>
<Style.Triggers>
<DataTrigger Binding="{Binding IsDragged}" Value="True">
<Setter Property="Background" Value="LightPink"/>
</DataTrigger>
<DataTrigger Binding="{Binding DragStatus}" Value="{x:Static Utils:DragEffects.Copy}">
<Setter Property="Background" Value="Lime"/>
</DataTrigger>
</Style.Triggers>
</Style>
The control style defines as a drag source the DragSource property of the data context of the item and synchronizes with the data context the properties of IsDragged and DragStatus. Triggers then make is pinkish when dragged and greenish when it can be dropped. Notice that this applies to the original item, while its representation is dragged, so you have a feedback of what is going on with the item right at the source.
I won't put here the ViewModels or the data items, since they are pretty much part of the business context, not the drag and drop. Just return DragEffects. All on the effects methods and you can drag anything anywhere, for example.
That's it, folks: drag and drop completely MVVM, without as much as writing an event handler or caring about the actual elements in the viewmodel. It would be even easier if you would allow references to WPF assemblies in the ViewModels, since you could also get the source elements and do stuff with them, but that wouldn't be much of an MVVM pattern, would it?
And here is the AdornerBase class, just a simple helper class:
/// <summary>
/// Basic adorner class that exposes simple Attach and Dettach methods
/// </summary>
public abstract class AdornerBase : Adorner
{
#region Instance fields
private bool mIsDettached;
#endregion
#region Properties
public bool IsDettached
{
get
{
return mIsDettached;
}
}
public AdornerLayer AdornerLayer
{
get;
private set;
}
#endregion
#region Constructors
protected AdornerBase(UIElement adornedElement)
: base(adornedElement)
{
mIsDettached = true;
}
#endregion
#region Public Methods
/// <summary>
/// Attach the adorner to the element's adorner layer
/// </summary>
public void Attach()
{
AdornerLayer = AdornerLayer.GetAdornerLayer(AdornedElement);
if (AdornerLayer != null)
{
AdornerLayer.Add(this);
mIsDettached = false;
}
}
/// <summary>
/// Dettach the adorner from the element's adorner layer
/// </summary>
public void Dettach()
{
AdornerLayer = AdornerLayer ?? AdornerLayer.GetAdornerLayer(AdornedElement);
if (AdornerLayer != null)
{
AdornerLayer.Remove(this);
mIsDettached = true;
}
}
#endregion
}
<system.net>
<defaultProxy>
<proxy
usesystemdefault = "False"
bypassonlocal = "False"
/>
</defaultProxy>
</system.net>
System.Configuration.ConfigurationErrorsException occurredand here is the second:
Message=Error creating the Web Proxy specified in the 'system.net/defaultProxy' configuration section.
Source=System
BareMessage=Error creating the Web Proxy specified in the 'system.net/defaultProxy' configuration section.
Line=0
StackTrace:
at System.Net.Configuration.DefaultProxySectionInternal.GetSection()
at System.Net.WebRequest.get_InternalDefaultWebProxy()
at System.Net.HttpWebRequest..ctor(Uri uri, ServicePoint servicePoint)
at System.Net.HttpRequestCreator.Create(Uri Uri)
at System.Net.WebRequest.Create(Uri requestUri, Boolean useUriBase)
at System.Net.WebRequest.Create(String requestUriString)
InnerException: System.Runtime.InteropServices.SEHException
Message=External component has thrown an exception.
Source=System
ErrorCode=-2147467259 (0x80004005)
StackTrace:
at System.Net.UnsafeNclNativeMethods.OSSOCK.WSASocket(AddressFamily addressFamily, SocketType socketType, ProtocolType protocolType, IntPtr protocolInfo, UInt32 group, SocketConstructorFlags flags)
at System.Net.Sockets.Socket.InitializeSockets()
at System.Net.NetworkAddressChangePolled..ctor()
at System.Net.AutoWebProxyScriptEngine.AutoDetector.Initialize()
at System.Net.AutoWebProxyScriptEngine.AutoDetector.get_CurrentAutoDetector()
at System.Net.AutoWebProxyScriptEngine..ctor(WebProxy proxy, Boolean useRegistry)
at System.Net.WebProxy.UnsafeUpdateFromRegistry()
at System.Net.WebProxy..ctor(Boolean enableAutoproxy)
System.Runtime.InteropServices.SEHException occurred.
Message=External component has thrown an exception.
Source=System
ErrorCode=-2147467259
StackTrace:
at System.Net.UnsafeNclNativeMethods.OSSOCK.WSASocket(AddressFamily addressFamily, SocketType socketType, ProtocolType protocolType, IntPtr protocolInfo, UInt32 group, SocketConstructorFlags flags)
at System.Net.Sockets.Socket.InitializeSockets()
at System.Net.IPAddress.InternalParse(String ipString, Boolean tryParse)
at System.Net.NetworkInformation.IpAddrString.ToIPAddressCollection()
at System.Net.NetworkInformation.SystemIPGlobalProperties.GetFixedInfo()
at System.Net.NetworkInformation.SystemIPGlobalProperties.get_FixedInfo()
at System.Net.NetworkInformation.SystemIPGlobalProperties.get_HostName()
at System.Net.NclUtilities.GuessWhetherHostIsLoopback(String host)
at System.Net.ServicePoint.get_ConnectionLimit()
at System.Net.ConnectionGroup..ctor(ServicePoint servicePoint, String connName)
at System.Net.ServicePoint.FindConnectionGroup(String connName, Boolean dontCreate)
at System.Net.ServicePoint.SubmitRequest(HttpWebRequest request, String connName)
at System.Net.HttpWebRequest.SubmitRequest(ServicePoint servicePoint)
at System.Net.HttpWebRequest.GetResponse()
public static DependencyProperty MyAttachedCollectionProperty =
DependencyProperty.RegisterAttached("MyAttachedCollectionInternal",
typeof (ObservableCollection<MyItem>),
typeof (ParentClass),
new PropertyMetadata(null));
public static void SetMyAttachedCollection(UIElement element, ObservableCollection<MyItem> value)
{
if (element != null)
{
element.SetValue(MyAttachedCollectionProperty, value);
}
}
public static ObservableCollection<MyItem> GetMyAttachedCollection(UIElement element)
{
ObservableCollection<MyItem> collection =
(ObservableCollection<MyItem>) element.GetValue(MyAttachedCollectionProperty);
if (collection == null)
{
collection = new ObservableCollection<MyItem>();
SetMyAttachedCollection(element, collection);
}
return collection;
}
#region Using directives
using System.Collections.Generic;
using System.ComponentModel;
using System.Windows;
using System.Windows.Controls;
using System.Windows.Markup;
#endregion
namespace BestPractices
{
/// <summary>
/// Base class for ControlTemplate transforming classes
/// </summary>
public abstract class BaseTemplateTransformer : BaseControlTransformer
{
#region Public Methods
/// <summary>
/// Clones the ControlTemplate of a control and allows for its manipulation
/// </summary>
/// <param name="control"></param>
public override void Transform(Control control)
{
ControlTemplate template = control.Template;
if (template == null)
{
return;
}
// create new template
ControlTemplate newTemplate = new ControlTemplate(template.TargetType);
// copy the resources
foreach (object key in template.Resources.Keys)
{
newTemplate.Resources.Add(key, template.Resources[key]);
}
//get the VisualTree factory from the original template content
DependencyObject content = template.LoadContent();
newTemplate.VisualTree = OnGetElementFactory(content);
// copy the triggers
foreach (TriggerBase trigger in template.Triggers)
{
newTemplate.Triggers.Add(trigger);
}
// allow for template manipulation
OnBeforeSeal(newTemplate);
// seal the template and set it back
newTemplate.Seal();
control.Template = newTemplate;
}
/// <summary>
/// Creates a custom ControlTransformFactory for the content object.
/// Override in order to replace elements in the initial template.
/// </summary>
/// <param name="content"></param>
/// <returns></returns>
public virtual ControlTransformFactory OnGetElementFactory(DependencyObject content)
{
if (content == null)
{
return null;
}
// use the object type
ControlTransformFactory factory = new ControlTransformFactory(content, this);
return factory;
}
/// <summary>
/// Returns a safe value for setting on the control
/// </summary>
/// <param name="item">The value from the template</param>
/// <returns></returns>
public virtual object GetSafeValue(object item)
{
return getSafeValue((dynamic) item);
}
/// <summary>
/// Returns true if a property needs to be saved and set when the control loads.
/// Defaults to false, except for ColumnDefinitions and RowDefinitions
/// </summary>
/// <param name="propertyDescriptor"></param>
/// <returns></returns>
public virtual bool MustSetProperty(PropertyDescriptor propertyDescriptor)
{
return sMustSetProperties.Contains(propertyDescriptor.Name);
}
#endregion
#region Protected Methods
/// <summary>
/// Allows for the manipulation of a control template
/// </summary>
/// <param name="newTemplate"></param>
protected virtual void OnBeforeSeal(ControlTemplate newTemplate)
{
}
#endregion
#region Statics
private static readonly List<string> sMustSetProperties = new List<string>
{
"ColumnDefinitions",
"RowDefinitions"
};
/// <summary>
/// Transforms a DependencyObject tree into a FrameworkElementFactory tree
/// </summary>
/// <param name="content"></param>
/// <returns></returns>
public static ControlTransformFactory CreateElementFactory(DependencyObject content)
{
if (content == null)
{
return null;
}
// use the object type
ControlTransformFactory factory = new ControlTransformFactory(content);
return factory;
}
/// <summary>
/// default return the same value
/// </summary>
/// <param name="value"></param>
/// <returns></returns>
private static object getSafeValue(object value)
{
return value;
}
/// <summary>
/// try to clone ColumnDefinition objects
/// </summary>
/// <param name="columnDefinition"></param>
/// <returns></returns>
private static object getSafeValue(ColumnDefinition columnDefinition)
{
return clone(columnDefinition);
}
/// <summary>
/// try to clone RowDefinition objects
/// </summary>
/// <param name="rowDefinition"></param>
/// <returns></returns>
private static object getSafeValue(RowDefinition rowDefinition)
{
return clone(rowDefinition);
}
/// <summary>
/// Clones an item via Xaml write/parse
/// </summary>
/// <param name="element"></param>
/// <returns></returns>
private static object clone(object element)
{
string str = XamlWriter.Save(element);
return XamlReader.Parse(str);
}
#endregion
}
}
#region Using directives
using System;
using System.Collections;
using System.Collections.Generic;
using System.ComponentModel;
using System.Reflection;
using System.Windows;
using System.Windows.Markup.Primitives;
using System.Windows.Media;
#endregion
namespace BestPractices
{
/// <summary>
/// FrameworkElementFactory used in control template transformers
/// </summary>
public class ControlTransformFactory : FrameworkElementFactory
{
#region Nested
internal class NoTemplateTransformer : BaseTemplateTransformer
{
}
#endregion
#region Instance fields
private readonly BaseTemplateTransformer mTemplateTransformer;
private RoutedEventHandler mLoadHandler;
private List<MarkupProperty> mSimpleProperties;
#endregion
#region Properties
/// <summary>
/// List of non dependency properties that will be set when the control loads
/// </summary>
protected List<MarkupProperty> SimpleProperties
{
get
{
if (mSimpleProperties == null)
{
mSimpleProperties = new List<MarkupProperty>();
}
return mSimpleProperties;
}
}
#endregion
#region Constructors
public ControlTransformFactory(DependencyObject content,
BaseTemplateTransformer templateTransformer = null)
: base(content.GetType())
{
mTemplateTransformer = templateTransformer ?? new NoTemplateTransformer();
// set its name
string name = content.GetValue(FrameworkElement.NameProperty) as string;
if (!string.IsNullOrWhiteSpace(name))
{
Name = name;
}
// copy the properties
foreach (MarkupProperty propertyItem in getProperties(content))
{
SetProperty(propertyItem);
}
// do it recursively
int count = VisualTreeHelper.GetChildrenCount(content);
for (int i = 0; i < count; i++)
{
DependencyObject child = VisualTreeHelper.GetChild(content, i);
AppendChild(mTemplateTransformer.OnGetElementFactory(child));
}
}
#endregion
#region Public Methods
/// <summary>
/// SetValue that uses reflection to force DependencyProperties that were registered as read only
/// </summary>
/// <param name="dependencyProperty"></param>
/// <param name="value"></param>
/// <param name="forceSetReadOnly"></param>
public void SetValue(DependencyProperty dependencyProperty, object value,
bool forceSetReadOnly = false)
{
if (!forceSetReadOnly)
{
base.SetValue(dependencyProperty, value);
}
else
{
forceSetValue(dependencyProperty, value);
}
}
/// <summary>
/// Sets a value from a MarkupProperty object.
/// Dependency properties will be set via SetValue and the others via a Loaded handler on the object
/// </summary>
/// <param name="propertyItem"></param>
public void SetProperty(MarkupProperty propertyItem)
{
DependencyProperty property = propertyItem.DependencyProperty;
if (property == null)
{
setSimpleProperty(propertyItem);
}
else
{
object value = propertyItem.Value;
if (value == DependencyProperty.UnsetValue)
{
return;
}
SetValue(property, value, property.ReadOnly);
}
}
#endregion
#region Private Methods
/// <summary>
/// Force set value in the factory, even if the property is ReadOnly
/// </summary>
/// <param name="dp"></param>
/// <param name="value"></param>
private void forceSetValue(DependencyProperty dp, object value)
{
object resourceKey = getResourceKey(value);
if (resourceKey == null)
{
updatePropertyValueList(dp, value is TemplateBindingExtension
? "TemplateBinding"
: "Set", value);
}
else
{
updatePropertyValueList(dp, "Resource", value);
}
}
/// <summary>
/// Invoke the private UpdatePropertyValueList on the factory
/// </summary>
/// <param name="dp"></param>
/// <param name="propertyValueTypeName"></param>
/// <param name="value"></param>
private void updatePropertyValueList(DependencyProperty dp,
string propertyValueTypeName, object value)
{
object propertyValueType = Enum.Parse(sPropertyValueTypeType, propertyValueTypeName);
sUpdatePropertyValueListProperty.Invoke(this, new[] {dp, propertyValueType, value});
}
/// <summary>
/// Set a property to have its value set at load time
/// </summary>
/// <param name="markupProperty"></param>
private void setSimpleProperty(MarkupProperty markupProperty)
{
if (markupProperty.PropertyDescriptor == null)
{
return;
}
if (!mTemplateTransformer.MustSetProperty(markupProperty.PropertyDescriptor))
{
return;
}
SimpleProperties.Add(markupProperty);
if (mLoadHandler == null)
{
mLoadHandler = new RoutedEventHandler(simplePropertyHandler);
AddHandler(FrameworkElement.LoadedEvent, mLoadHandler);
}
}
/// <summary>
/// Handler on the Loaded event of the control
/// </summary>
/// <param name="sender"></param>
/// <param name="args"></param>
private void simplePropertyHandler(object sender, RoutedEventArgs args)
{
foreach (MarkupProperty propertyItem in SimpleProperties)
{
PropertyDescriptor propertyDescriptor = propertyItem.PropertyDescriptor;
if (propertyDescriptor == null || propertyItem.Value == null)
{
continue;
}
if (propertyDescriptor.IsReadOnly)
{
IList list = propertyItem.Value as IList;
if (list != null)
{
IList destinationList = propertyDescriptor.GetValue(sender) as IList;
if (destinationList != null)
{
foreach (object item in list)
{
destinationList.Add(mTemplateTransformer.GetSafeValue(item));
}
}
else
{
//shouldn't happend
}
}
else
{
// what now?
}
}
else
{
propertyDescriptor.SetValue(sender, mTemplateTransformer.GetSafeValue(propertyItem.Value));
}
}
FrameworkElement element = (FrameworkElement) sender;
element.RemoveHandler(FrameworkElement.LoadedEvent, mLoadHandler);
}
#endregion
#region Statics
static ControlTransformFactory()
{
// Get the types and methods that will be used in Reflection scenarios
sUpdatePropertyValueListProperty =
typeof (FrameworkElementFactory).GetMethod("UpdatePropertyValueList",
BindingFlags.NonPublic | BindingFlags.Instance);
sPropertyValueTypeType =
typeof (FrameworkElementFactory).Assembly.GetType("System.Windows.PropertyValueType");
}
private static readonly MethodInfo sUpdatePropertyValueListProperty;
private static readonly Type sPropertyValueTypeType;
/// <summary>
/// get the properties set on a DependencyObject
/// </summary>
/// <param name="content"></param>
/// <returns></returns>
private static IEnumerable<MarkupProperty> getProperties(DependencyObject content)
{
MarkupObject markupObject = MarkupWriter.GetMarkupObjectFor(content);
return markupObject.Properties;
}
/// <summary>
/// Get the ResourceKey property from an object, if it exists
/// </summary>
/// <param name="target"></param>
/// <returns></returns>
private static object getResourceKey(object target)
{
if (target == null)
{
return null;
}
Type type = target.GetType();
PropertyInfo property = type.GetProperty("ResourceKey");
if (property == null)
{
return null;
}
return property.GetValue(target, new object[] {});
}
#endregion
}
}
private byte[] getPublicKey(FileInfo fi)
{
using (
FileStream stream = File.Open(fi.FullName,
FileMode.Open,
FileAccess.Read,
FileShare.ReadWrite))
{
byte[] arr = new byte[4];
stream.Seek(8, SeekOrigin.Begin);
stream.Read(arr, 0, arr.Length);
var publicKeyLength = BitConverter.ToInt32(arr, 0);
arr = new byte[publicKeyLength];
stream.Seek(16, SeekOrigin.Begin);
stream.Read(arr, 0, arr.Length);
return arr;
}
}
private string getExtensionId(byte[] publicKey)
{
SHA256 sha = SHA256.Create();
publicKey = sha.ComputeHash(publicKey);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 16; i++)
{
byte b = publicKey[i];
char ch = (char)('a' + (b >> 4));
sb.Append(ch);
ch = (char)('a' + (b & 0xF));
sb.Append(ch);
}
return sb.ToString();
}
using System;
using System.IO;
using System.Security.Cryptography;
using System.Text;
using System.Web.Script.Serialization;
using SevenZip;
namespace ChromeExtensionInstaller
{
internal class CrxPack
{
#region Instance fields
private byte[] mContent;
private SevenZipExtractor mExtractor;
private dynamic mManifest;
private Uri mUri;
private string mPath;
#endregion
#region Properties
public Exception InvalidReason
{
get;
private set;
}
public bool IsValid
{
get;
private set;
}
private dynamic Manifest
{
get
{
if (mManifest == null)
{
FileInfo fi = new FileInfo(mUri.AbsolutePath);
mManifest = getManifest(fi);
}
return mManifest;
}
}
public string Id
{
get
{
return getExtensionID();
}
}
public string Name
{
get
{
return Manifest.name as string;
}
}
public string Version
{
get
{
return Manifest.version as string;
}
}
public string Path
{
get
{
return mPath;
}
}
#endregion
#region Constructors
public CrxPack(string path)
{
mPath = path;
try
{
checkPath(path);
IsValid = true;
}
catch (Exception ex)
{
IsValid = false;
InvalidReason = ex;
}
}
#endregion
#region Private Methods
private void checkPath(string path)
{
mUri = ExtensionHelper.GetUri(path);
if (mUri == null)
{
throw new Exception(string.Format("Parameter is not a valid URI ({0})", mPath));
}
mPath = mUri.AbsolutePath;
if (!mUri.IsFile && !mUri.IsUnc)
{
throw new Exception(string.Format("Only file and local network paths are acceptable ({0})",
mPath));
}
DirectoryInfo di = new DirectoryInfo(mPath);
if (di.Exists)
{
throw new Exception(string.Format(
"Loading extensions from folders is not implemented ({0})", mPath));
}
FileInfo fi = new FileInfo(mPath);
if (!fi.Exists)
{
throw new Exception(string.Format("The file does not exist ({0})", mPath));
}
if (fi.Extension.ToLower() != ".crx")
{
throw new Exception(string.Format("The file extension must be a .crx file ({0})", mPath));
}
try
{
mExtractor = getExtractor(fi);
if (mExtractor.Check())
{
return;
}
}
catch (Exception ex)
{
throw new Exception(
string.Format("The file could not be read as a valid .crx file ({0})", mPath), ex);
}
throw new Exception(string.Format("The file could not be read as a valid .crx file ({0})",
mPath));
}
private SevenZipExtractor getExtractor(FileInfo fi)
{
byte[] arr;
using (
FileStream stream = File.Open(fi.FullName, FileMode.Open, FileAccess.Read,
FileShare.ReadWrite))
{
arr = new byte[fi.Length];
mContent = arr;
stream.Read(arr, 0, arr.Length);
}
// force PkZip signature
arr[0] = 0x50;
arr[1] = 0x4B;
arr[2] = 0x03;
arr[3] = 0x04;
MemoryStream ms = new MemoryStream(arr);
return new SevenZipExtractor(ms);
}
private string getExtensionID()
{
int length = readInt(8);
byte[] bytes = readBytes(16, length);
SHA256 sha = SHA256.Create();
bytes = sha.ComputeHash(bytes);
StringBuilder sb = new StringBuilder();
for (int i = 0; i < 16; i++)
{
byte b = bytes[i];
char ch = (char) ('a' + (b >> 4));
sb.Append(ch);
ch = (char) ('a' + (b & 0xF));
sb.Append(ch);
}
return sb.ToString();
}
private int readInt(int index)
{
byte[] bytes = readBytes(index, 4);
return BitConverter.ToInt32(bytes, 0);
}
private byte[] readBytes(int index, int length)
{
byte[] bytes = new byte[length];
Array.Copy(mContent, index, bytes, 0, length);
return bytes;
}
private object getManifest(FileInfo fi)
{
SevenZipExtractor extractor = getExtractor(fi);
string json;
using (MemoryStream ms = new MemoryStream())
{
extractor.ExtractFile("manifest.json", ms);
ms.Seek(0, SeekOrigin.Begin);
StreamReader sr = new StreamReader(ms);
json = sr.ReadToEnd();
}
JavaScriptSerializer serializer = new JavaScriptSerializer();
serializer.RegisterConverters(new[] {new DynamicJsonConverter()});
return serializer.Deserialize(json, typeof (object));
}
#endregion
}
}
Windows Registry Editor Version 5.00
[HKEY_LOCAL_MACHINE\SOFTWARE\Microsoft\Cryptography\Defaults\Provider\Microsoft Enhanced RSA and AES Cryptographic Provider]
"Image Path"="rsaenh.dll"
"Type"=dword:00000018
"SigInFile"=dword:00000000